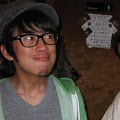
Forward 2 Notes - Part 2
This post is a continuation from my earlier post, Foward 2 Notes - Part 1
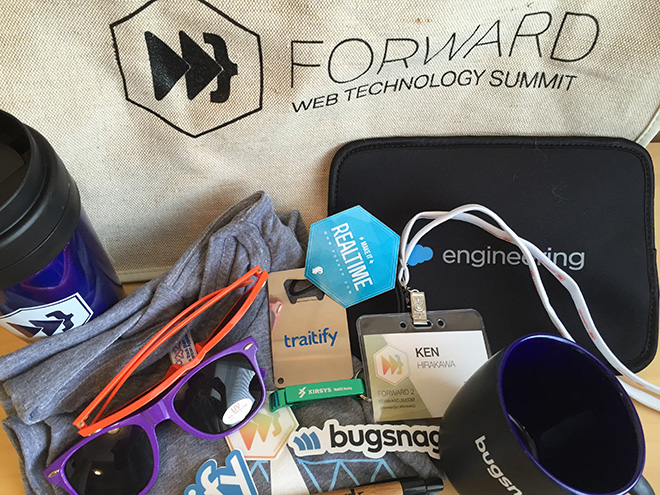
Here are the talks I attended:
- Keynote by Karolina Szczur and Sarah Groff-Paermo
- The Better Parts, Douglas Crockford
- What the… JavaScript?, Kyle Simpson
- We Will All Be Game Programmers, Hunter Loftis
- No More Tools, Karolina Szczur
- Developing High Performance Websites and Modern Apps with JavaScript and HTML5, Doris Chen
- Love & Node, Sarah Groff-Palermo
- Developer Led Innovation, James Sacra
- Choosing a JavaScript Framework, Pam Selle
- Test-driven client-side apps, Pete Hodgson
This post will have notes on the remaining talks starting with Hunter Loftis. Enjoy!
We Will All Be Game Developers, Hunter Loftis
3 weeks to build out an FPS game in HTML5 for an internationally releasing album.
Issues
- Budget (cheap), because the marketing people found Hunter really late, and so they had little left
- 3 weeks
- Technical constraints (must work on iPhone, iPad, Android & Desktop!)
Sure! sounds like an awesome project. Why not help these “no-name” artists. But… Apparently the guy behind the album is a big artist in the UK, and Hunter was the no-name artist. Pressure! For the next three weeks, no sleep.
“Optimism is an occupational hazard of programming” - Kent Beck
Demo
- Looks a lot like minecraft
- The cool part is that if you get lost in the maze, it plays the sound track “Lost”
- Got a Kotaku article out of it as well. Now technically a “game developer”
Why should You Care?
- Application interfaces are becoming more like games
- JS developers aren’t strong in game development. But the tech industry is moving towards game like interfaces with Holograph, VR, leap motion, etc
- Building a simple game like pong is a lot harder than most complicated applications
3 Ideas from Game Dev for Better User Interfaces
Let’s steal from game dev community
1. Minimize and isolate state.
- State is the root of all evil
- JS makes it easy to add type to anything
- Game devs don’t have much luxury. Compare Todolists VS Call of Duty. There’s much more state to manage and yet they get 60 fps. Minimizing state is important for complex applications
For a simple game of a guy walking around, we might need to keep track of:
- direction
- XY coordinates
- velocity
- If he runs fast, kick off dust…
We can all capture all that with just these:
function Player() {
this.x = [0, 0, 0];
this.y = [0, 0, 0];
this.interval
this.distance
}
And a set of pure functions. Given an input -> Always the same output.
var velocity = this.getVelocity(state.x, state.y, state.interval);
var direction = this.getDirection(velocity);
var pose = this.getPose(state.distance);
var frame = this.getFrame(direction, post);
No side effects. The point is to figure out where the state is, and then use pure functions to derive the rest.
2. Enforce deterministic rendering (frames should be independent)
function render(seconds) {
this.renderer.render(seconds, this.getState());
}
It would be a lot easier to do undo / redo if we kept a history of states, instead of modifying a state object directly. (again, FP and immutability is a big hot topic at Forward.)
Keep state independent of rendering. React is great at doing this. React works like a game engine and supports isomorphic JS
3. Separating rendering and simulation
Simulation leads to good animation.
The pursuit of 60fps
Compared to:
- cinema: 24fps
- with blur: 18fps
- fighter pilos: 220 fps!!
If you’re delivering any online experience, optimize for 60 fps
setInterval(frame, 0)
is badsetInterval(frame, 17)
is better, but does not guarantee that the frame method runs at the next framerequestAnimationFrame(frame)
is better, but is not fully guaranteed because you can still drop frames. If that virus scanner runs while your game is running, your game might drop frames.
Instead, what you want to do is decouple rendering and simulation. If you drop a frame, you track it. For every frame you drop, you simulate it. Drop 2 frames, simulate it twice. This way, you get the same exact behavior, regardless of dropped frames.
My favorite bug
Ken’s Thoughts
What he said about TODOMVC makes a lot of sense… We fuss over which framework to use to build such a simple TODOMVC, yet game developers are building complex worlds and game mechanics all in 60 fps.
No More Tools, Karolina Szczur
Have we reached a tipping point with too many tools? A look at up-to-date front-end tooling and better ideas for teamwork
Tools
Tool - we have a plethora of tools to use to help us make our lives easier, but is that always the case?
“Creatives aren’t good at their art because of their tools; their talent stems from the skills and knowledge they’ve acquired while using their tools” - The Good Creative - Paul Jarvis
But tools are still good to use. Which frameworks / tools are good and what will eventually break our heart?
Things to think about
- Simplicity. Two types of simple. Visual simple - does it look simple to use? Operational simplicity - is it easy to use
- Does it allow us to single-thread our attention? Most crucial skill is to not multi-task, but single-threading our attention.
- Complexity. The time it takes to learn something. Tesler’s Law of Conservation of Simplicity. The strive for simplicity is superficial because at times, complexity is necessary. Its a fact of the world and simplicity is in the mind.
Automation
Automation gives us time to work on problems that cannot be delegated to scripts
Tools
- Autoprefixer - worry-free vendor prefixing
- Linting - check for bad patterns
- Media Optimisation - strip unnecessary bytes.
- Minification - strip unnecessary characters
- Code bloat - might might not be useful. Search and remove unused declarations
- Testing performance - find specific elements hindering performance
- Debugging layout issues - find problems with the box model. pesticide.io
- GUIs for automation - Hammer format, codekit, livereload
NPM
- NPM is a way to run scripts as well. Its a native ecosystem for task automation.
- Some people think npm for front-packaging isn’t good enough, but “npm loves you, front-enders, and we are about your use cases.”
- bit.ly/leveldb-example
- bit.ly/npm-task-automation
Gulp & Grunt
- Alternatives to make
- Gulp might be better for node people because it uses streams.
- Grunt is more configuration over coding.
- Which is better? Matter of preference. She seems to prefer Gulp
Make
- Why not use make? People tend to forget about it, but make is quite powerful
- bit.ly/buidling-static
When to do what?
- When should we minify code?
- A Case for minifying and committing the minified code. -> Allows people without minification tools to use your scripts
Collaboration
- The tools are there to empower ourselves.
- Collaboration happens when our own set of biases doesn’t cloud judgement. Tools are a way
Developing High Performance Websites and Modern Apps with JS and HTML5, Doris Chen
Game - How to make it run faster
This talk is about the principles to improving the JS performance of your app
Today, we’ll improve a game called High Five Yeah. The more you get high fives, the more points you get.
Components and control flow of the game
- Matrix of players
- Each player has a set of directions
- On touch select a player
- Generate a list of neighbors to rotate
- Rorate the player
- Repeat from step 4
The game allocates lots of memory if you increase the size of the grid.
Always measure
- profile the game.
- Define the criteria of what “fast enough” is first. You can use IE, but feel free to use other tools.
- IE developer tool has a tab called responsiveness. It gives you a chart of FPS and CPU usage.
- The three important measurements - CPU (tied to battery life), GPU, and FPS.
- Similar to chrome, IE dev tools gives you color coded results - yellow for layout, green for css style calculations, etc.
- The goal is to have a flat 60 fps, no dips in the charts.
Principle 1: Memory usage - Stay Lean
- Every call to new or implicit memory allocation reserves GC memory - allocations are cheap until current pool is exhausted. Every time the GV happens, we will see some obvious pauses
- When pool is exhausted, engines force a collection
Best practices for staying lean
- Avoid unnecessary object creation
- Look at object creation pattern. Use object pools when possible.
- Be aware of allocation patterns, such as Setting closures to event handlers.
Principle 2: Use Fast Object Types and Manipulations
- In JS the order of a hash map matters. It is better to keep the “shape” the same. For example.
var a = {}; a.north = 1; a.south = 0;
andvar b = {}; b.south = 0; b.north = 1
do not share the same “shape” - Avoid creating slower property bags. Don’t use getters and setters for perf critical paths. For example, its probably better to use
this.n = north
instead of doing
Object.defineProperty(this, "n", {
get: function () { return nVal;}
})
- Use simple objects for perf critical paths
- Never use
delete
, it is sloooow
Principle 3: Best Practices for Fast Arithmetic
- Use 31-bit integer math when possible. 31 will go onto the stack, but a 32 float will not. It needs to move to a slower, long term storage.
- Avoid floats if they are not needed
- Design for type specialized arithmetic. Try to separate floating arithmetic from integer arithmetic
Principle 4: Do Less Work
Avoid chattiness with the DOM
BAD
document.body.get.getElementById("id").classList.removeClass(oldClass)
document.body.get.getElementById("id").classList.addClass(newClass)
GOOD
var el = document.body.get.getElementById("id").classList;
el.removeClass(oldClass);
el.addClass(newClass)
If you know a value is a integer, such as when this.borderSize = domelement.getBorderSize
, it is better to parseInt
it. 25% better performance in marshaling.
Paint as much as your users can see, which is 60 FPS
- BAD
setTimeout(animate, 0)
<- this is more work because its trying to animate too many times. - BETTER
requestAnimationFrame(animate)
<- let the browser determine when to animate
Key Take Away
For super performance critical code, we can do a lot of micro optimizations to make performance better. Better performance = longer battery life,
Love & Node, Sarah Groff-Palermo
This is the second part to her key note about relatables - objects that allow us to explore the relationship between people and objects
Creating Relatability
How do we create a robot that is relatable?
There are 6 ways
- Give it a face. From a very young age, humans are learned to detect faces. @facepics
- Sense of agency
- Social behavior - teaching objects to remember.
- Make the robot unpredictable. gives the feeling of personality
- Creating a sense of helplessness. Helps people feel less lonely.
- Objects that mimic us. If I turn my head and it turns its head then we like it.
Process
How does one create a robot? Her process.
Take input -> Do something with it (the gear) -> Output
1. Take input
- Information to take in, who is nearby, how are you feeling, which books haven’t I read?
- Tools to use - proximity (IR and ultrasonic), climate temp and humidity, ambient (light and sound), bluetooth, gas, accelerometer, motion, touch (capacitive and pressure) sensors.
2. The gear
- Turn inputs into outputs. Tessel, Arduino, and RPi.
- Arduino - C derived Arduino language. Node via Johnny 5, DIY / Sheilds Tessel - Node, wifi builtin, high-level modules
- Both have awesome communities
3. Outputs
Possible outputs
- regular LEDS
- addressable LEDS
- motors
- servors speakers
Projects
- FriendBot - Built at SFPC
- Neo-Neglect - Books that have emotion. If it isn’t read for a while, it screams “You’re a monster!”
- Spiny - Actually a failure, but its always a good thing to love all our monsters
- Deluge - The feelings of being overwhelmed. Senses BT signals in a room and visualizes it
Questions and Demos
Developer Led Innovation, James Sacra
Failure
- Developed new prototype for voice recognition in 3 months and ready to blow manager minds away
- Got embarrassed at a call with sales people. Turns out users didn’t want the prototype he developed.
How did he fail?
- He didn’t understand the key problem
- He didn’t explain the solution
- He didn’t gain traction
Steps to Getting Your Idea Heard
Step 0: Need a friend (ally). - Someone to support your ideas. They can help you push your ideas from a unique perspective. - Who should you befriend? Fellow developers, technical managers, business support, etc. Grab them over lunch, talk to them
Step 1: What’s the problem?
- In order to innovate you must understand the problem. Its not about, oh I want to use D3!
- Quantify - 20% of customers are negatively impacted? What is the probem and how do you quantify it?
- At the same time, its important to keep developers happy and NOT use a technology from 10 years ago.
Step 2: Innovate
- Think tank! Innovate. Hackathons. This can even be a 3, 4 hour meeting with key people.
- Prototyping - gives you answers you need in next step, a great demo, and flushes out a lot problems
Step 3: Define the solution
- Quantify the solution - “We developed a prototype in half the time than we could in the existing framework”
- Keyword is “we”.
- People usually don’t realize minor performance issues until they see numbers.
Step 4: Pitch
- Make a presentation. Include your assessment of the problem, impact to the business, and the solution. It will help you think through questions that wil be asked and might even lead you to do more research
- Technical initiatives - at this point you should have a strong case for why you idea needs to be heard. And if you’re not being heard, go back to step 0
Troubleshooting
- “No matter what I do… I can’t get someone to listen”. This is just an excuse. You haven’t put in the effort to be heard.
- “We don’t have enough available resources to innovate”. No, you do. During lunch or meetings or whatever, find the time
Key Take Away
Don’t be afraid to fail, that is the only way to succeed
Choosing a Javascript Framework, Pam Selle
There’s a book!
Spoiler: I will not tell you what to do. The hope is for you to make informed decisions for a project
What is a JS framework?
- Most framework were built in 2009 / 2010 - “Oh I found that I was building the same thing over and over again for consultants so I figured, I should build my own!”
- Frameworks are good because you shouldn’t have to write boilerplate code.
- Ask yourself, what’s our 15% (the thing that is not boilerplate, and makes your app special) Then find the framework that makes you go fast
Backbone
- Backbone comes from a Rails world
- It’s the “core” of an application
- It’s un-opiniated. Generally more flexible. e.g. incorporating other libraries. This is both a strength and a weakness
- Has models, views, collections, events, and routing
- It’s quite intuitive to get started
Angular
- Fastest growing JS framework…
- Directives! and Google!
- Strongly defined building components (directives, controllers, services)
- Dependency injection
- Two way bindings
- Karma and protractor for testing
- No dependencies
- Strengths - once you know what you are doing, there’s less to write. Long feature list. Module-friendly. Google backing
- Weaknesses - not as battle tested. High lock-in with writing behavior in markup. Skeptical about Google backing. 2.0 drops backwards compatibility
- Has Modules, Directives, Services, Controllers, and other components like Filters, animations, i18n, l10n
Ember
- Most complete JS framework (not necessarily the best). Used for really intense client side applications
- Built completely on modular open source components
- Community super-powered
- Very strongly defined MVC components
- two way data binding
- Ember components (like directives or web components)
- dependency injection
- Routing
- Dependencies - ember-data, jQuery, handlebars
- Strengths - convention driven like rails, the community!
- Weaknesses - Sometimes TOOO much information, the “right” way to do things = difficult to get up to speed quickly, lots of rules, not as pervasive as other two frameworks
Rising stars!
React
- React is like git diffs for browser rendering
- Super performant
- The view of MV*
PolymerJS
- Web components!
Evaluating frameworks
- TODOMVC
- bit.ly/lg6kDSS - ranking for spreadsheets
- Rank frameworks according to bsuiness, tehcnical, and team criteria
- DevTap - technological evaluations
Test Driven Client-side Apps, Pete Hogsdon
Test driven development
- Write a failing test
- Write code that makes it pass
- Refactor code (people miss this part a lot)
- Go back to 1
The whole point is to build out a test suite that gives you the safety net to go and refactor your code to make it better
What’s the Advantage of TDD?
- Feedback! TDD gives repeated, consistent, direction on a granular level as to if your code works as you specified it.
- Feedback on the design of your code. It makes you write code that is easier to test
- Easily testable code makes it easier to work with. “This code is hard to test” === “This code is hard to work with”
Live Coding - Example of TDD
- nxtbrt.com - single page app with list of BART departures.
- LIVE CODING - went through the above four steps to demonstrate power of TDD.
- We all want to rewrite a code base without any tests. We don’t make things better every time because we dont have the confidence to do it. TDD gives you that confidence.
- Feed back is rapid, focussed, and accurate.
Functional testing
- The feedback you get back with functional testing is not as helpful because the scope is larger with FT. Especially if the functional test is testing an app with external dependencies such as HTTP and DOM.
- The key principle with unit tests is that we test an isolated unit of code. We get faster, more accurate feedback.
- Doesn’t mean functional testing is bad though.
Key Take Away
TDD drives developers toward better design
Conclusion
And that’s it folks! Hope you got a glimpse of what Forward 2 was like. It will be interesting to look back to this post 10 years from now and see how JavaScript has moved forward. What I’d like to know is, will JavaScript still be the monopoly client-side language it is today? My gut feeling tells me that it will, and will be for a good long time.